Have you ever faced the frustration of a C# source generator that just won’t work? You’re not alone. Many developers find themselves stuck, scratching their heads over why their code isn’t generating as expected. It can feel like a roadblock in your project, slowing down progress and adding unnecessary stress.
In this article, you’ll discover common pitfalls and troubleshooting tips to get your source generator back on track. Whether it’s a misconfiguration or a missing dependency, we’ll help you identify the issue and provide practical solutions. By the end, you’ll feel more confident tackling source generator challenges and keep your projects moving forward.
Key Takeaways
- Understanding Source Generators: C# source generators automate code generation at compile time, reducing repetitive tasks and enhancing development efficiency.
- Common Issues: Misconfigurations in project files, invalid attributes, and namespace conflicts are typical reasons source generators may not function properly.
- Troubleshooting Techniques: Familiarize yourself with diagnostic tools in Visual Studio, utilize Roslyn analyzers for real-time feedback, and implement logging for better insight during the code generation process.
- Best Practices: Regularly update dependencies, ensure correct code annotations, and start with simple generators to build understanding before progressing to more complex implementations.
- Documentation as a Resource: Use Microsoft’s detailed documentation on source generators for coding best practices and to address common pitfalls effectively.
Overview of C# Source Generators
C# source generators are powerful tools that enable you to generate C# code at compile time. They help streamline code generation and reduce the need for repetitive coding tasks. By automating parts of the development process, you can focus on building robust applications.





Key Features of Source Generators
- Compile-time Code Generation: Source generators create additional code files during the compilation phase. This means you can produce new classes or methods without writing them manually.
- Integration with Roslyn: Built on the Roslyn compiler platform, source generators leverage existing C# features. You can analyze code, create new code, and maintain project consistency.
- Customization: You can create source generators tailored to specific project needs. This customization can enhance productivity by generating repetitive patterns or boilerplate code.
Common Use Cases
- Data Transfer Objects (DTOs): Automatically generate DTOs from data models to reduce manual coding.
- Serialization: Create serialization code for complex objects, simplifying data handling.
- Auto-implemented Interfaces: Generate interface implementations, minimizing code duplication.
Troubleshooting Common Issues
- Misconfigured Project Files: Ensure your project file includes necessary references and package dependencies. Check for the
Microsoft.CodeAnalysis.CSharp.SourceGenerators
package. - Invalid Attributes: Source generators rely on specific attributes. Missing or incorrectly set attributes can hinder their functioning.
- Namespace Conflicts: Verify that your generated code does not clash with existing namespaces. This conflict can lead to inaccessible types or methods.
- Start Simple: Begin with straightforward generators to familiarize yourself with the process. Incremental complexity can lead to better understanding.
- Leverage Documentation: Use Microsoft’s documentation for source generators as a valuable resource. It explains implementation details and offers coding best practices.
- Test Thoroughly: Conduct tests to validate the generated code’s behavior. Ensure it integrates correctly with your existing codebase.
Incorporating C# source generators can significantly enhance your coding efficiency, provided you avoid common pitfalls and implement best practices.
Common Issues With C# Source Generators
Several common issues can arise while working with C# source generators, impacting their functionality and effectiveness.
Misconfiguration of Project Settings
Misconfiguration often leads to source generators not being recognized or executed. Ensure the following:
- Target Framework: Your project must target a supported framework version compatible with the source generator.
- Project File Setup: Check if the
<GenerateAssemblyInfo>
tag in your .csproj file is set tofalse
. This prevents mixed metadata issues. - Package References: Ensure the necessary NuGet packages for the source generator are accurately listed in your project. Absent or incorrect versions can cause runtime failures.
- Build Configuration: Verify you’re building in the right configuration, such as Debug or Release, which can define where and how source generators execute.
Incompatible Compiler Versions
Using incompatible versions of the C# compiler can obstruct the intended behavior of source generators. Focus on these key points:
- Roslyn Compatibility: The version of the Roslyn compiler must align with your source generator dependencies. A mismatch might result in unexpected errors or no generated code.
- Update Visual Studio: Regularly update Visual Studio and any associated SDKs. Incompatibilities often arise with older versions of tools.
- Check Source Generator Requirements: Review documentation for any specific compiler or framework requirements related to the source generator you’re using.
Addressing these common issues reduces complications and enhances your experience with C# source generators.





Debugging C# Source Generator Problems
Debugging C# source generator issues requires a systematic approach. By employing the right tools and techniques, you can identify the problems quickly and effectively.
Tools and Techniques for Debugging
- Visual Studio Diagnostic Tools: Use the built-in diagnostic tools in Visual Studio. The Debug Output window reveals useful logs during the build process.
- Roslyn Analyzer and Code Fixes: Leverage Roslyn unless you’re already using it. Integrating Roslyn analyzers can provide real-time feedback on syntax or semantic errors.
- Logging in Source Generators: Implement logging directly in your source generator code. Use simple
Console.WriteLine
or a logging library to output essential information that helps track the code generation process. - Unit Testing: Create unit tests for your source generator. Make sure to test various inputs to ensure the generator functions as expected under all conditions.
- Review Documentation: Check official Microsoft documentation for updated guidelines. Resources on MSDN provide insights into common pitfalls and debugging strategies.
Analyzing Build Output
Analyzing build output helps pinpoint issues. Focus on the following steps:
- Build Output Window: Access the build output window in Visual Studio. Select the “Build” output and look for any warnings or errors related to your source generator.
- Check for Warnings: Look for warnings that might affect the source generation. Some might indicate missing or misconfigured attributes that could lead to incorrect behavior.
- Compare with Expected Output: Take the generated output and compare it to the expected result. Identifying discrepancies can lead you to the cause of the problem quickly.
- Review Project Configuration: Examine the project file for correct generator settings and attributes. Ensure that the source generator projects are included in the solution correctly.
- Explore Compiler Diagnostics: Enable additional compiler diagnostics to give greater clarity on errors involving source generators. This can help identify issues with specific syntax or semantic errors early in the process.
Using these techniques and tools will streamline your debugging process, helping you resolve issues effectively and enhancing your development experience with C# source generators.
Solutions to C# Source Generator Not Working
You can resolve the issue of C# source generators not working by following several steps. Consider these recommendations to troubleshoot effectively.
Updating Dependencies
Check your project’s dependencies regularly. Use the latest versions of NuGet packages related to source generators. This ensures compatibility with the current Roslyn compiler.





- Open the NuGet Package Manager: Access it from your project in Visual Studio.
- Review Installed Packages: Look for any outdated packages.
- Update Packages: Click on the update button for any packages that need updating. Ensuring all dependencies align with your target framework minimizes potential conflicts.
Checking Code Annotations
Code annotations play a crucial role in making source generators function smoothly. Validate that you’ve correctly applied annotations in your classes or methods.
- Review Required Attributes: Ensure you use relevant attributes like
[Generator]
in your source generator code. - Inspect Generated Types: Confirm that generated types and members follow expected protocols set by attributes.
- Resolve Namespace Conflicts: Ensure that namespaces do not overlap across your project. Unique namespaces can prevent unexpected behavior.
By applying these methods, fixing issues with C# source generators becomes manageable, leading to a smoother development experience.
Conclusion
Dealing with C# source generator issues can be frustrating but you’re not alone in this journey. By understanding common pitfalls and applying the troubleshooting tips shared, you can tackle these challenges head-on.
Remember to keep your project configurations in check and ensure your dependencies are up to date. Regularly testing your generated code and utilizing the right tools can make a world of difference.
With a little patience and practice, you’ll find that managing source generators becomes a smoother part of your development process. Stay curious and keep experimenting to enhance your coding efficiency. Happy coding!
Frequently Asked Questions
What are C# source generators?
C# source generators are tools that create C# code at compile time. They streamline development by automating repetitive tasks, helping developers focus on core logic rather than boilerplate code.
What problems do developers face with C# source generators?
Common problems include misconfigurations, missing dependencies, namespace conflicts, or using incompatible compiler versions. These issues can stall project progress if not addressed promptly.
How can I troubleshoot C# source generator issues?
Start by verifying project configurations, ensuring correct NuGet packages are included, and checking for compatibility with the targeted framework. Use tools like Visual Studio’s diagnostics for detailed insights.
What should I do if my source generator is not working?
Make sure your project files are properly configured, relevant attributes are applied correctly, and that your environment (such as SDKs and Visual Studio) is up to date. This often resolves many issues.
How can I improve my experience with C# source generators?
Regularly update your dependencies, carefully apply code annotations, and test generated code thoroughly. Following best practices and utilizing documentation can enhance your development experience significantly.
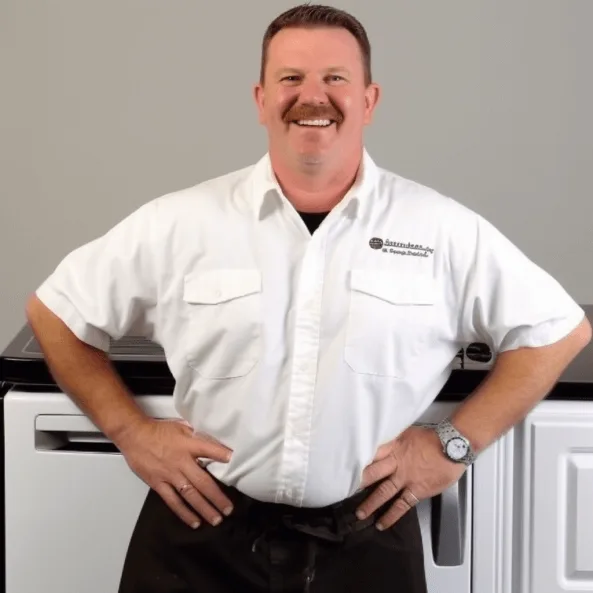
Hey, I’m Jake. I focus on cooling systems at Appliance Mastery, like fridges, freezers, and air conditioners.
I’ve worked in appliance repair for more than ten years and I’m certified through NASTeC. I’ve seen just about every fridge issue you can imagine.
My goal is to help you fix problems without stress. Whether it’s a freezer that won’t cool or an AC that keeps beeping, I’m here to walk you through it.