Have you ever faced the frustration of a JPA sequence generator that just won’t work? You’re not alone. Many developers encounter this issue, leading to wasted time and headaches. Whether you’re starting a new project or maintaining an existing one, a malfunctioning sequence generator can throw a wrench in your plans.
Key Takeaways
- Understanding JPA Sequence Generators: JPA sequence generators are essential for creating unique identifiers in applications, ensuring data integrity and efficiency.
- Common Reasons for Malfunction: Issues often arise from configuration errors, database compatibility problems, or application-specific issues affecting the JPA sequence generator’s functionality.
- Troubleshooting Steps: Verify entity configurations, check the existence of the database sequence, and debug application code to diagnose and resolve sequence generator issues effectively.
- Best Practices for Configuration: Ensure proper annotation usage, validate database sequences, choose appropriate allocation sizes, and maintain consistency in data types to prevent errors.
- Monitoring and Testing: Implement logging to track ID generation and conduct both unit and integration tests to validate the sequence generator’s performance and reliability under various conditions.
- Permission Checking: Confirm that the database user has adequate permissions to access the sequence, as insufficient access can lead to generator failures.
Understanding JPA Sequence Generator
JPA sequence generators provide a reliable way to generate unique identifiers in your application. These generators use database sequences that allow for efficient ID creation, essential for maintaining data integrity.
What Is a JPA Sequence Generator?
A JPA sequence generator defines a strategy for generating primary keys. You annotate your entity with @SequenceGenerator
, specifying a name and other parameters. The @GeneratedValue
annotation then indicates that the ID should be generated with the specified sequence. This setup ensures that each entity instance gets a unique identifier from the database sequence.
Example:
@Entity
public class MyEntity {
@Id
@GeneratedValue(strategy = GenerationType.SEQUENCE, generator = "my_seq_gen")
@SequenceGenerator(name = "my_seq_gen", sequenceName = "my_sequence", allocationSize = 1)
private Long id;
// other fields and methods
}
Common Use Cases for JPA Sequence Generators
You typically use JPA sequence generators in various scenarios:
- High-Volume Applications: In applications with high data insertion rates, sequences enhance performance by reducing contention.
- Distributed Systems: When working in distributed environments, sequences ensure unique IDs across different nodes.
- Database Portability: Sequences offer a standardized way to handle IDs that work across different database systems, making migration easier.
Using JPA sequence generators promotes consistency and efficiency in managing your entity IDs, which is crucial for your application’s reliability.
Reasons Why JPA Sequence Generator Is Not Working
Issues with a JPA sequence generator often stem from several common sources. Understanding these reasons can help you diagnose and resolve problems effectively.
Configuration Errors
Configuration errors often cause the JPA sequence generator to malfunction. Check the following settings:
- @SequenceGenerator Annotation: Ensure the correct parameters are specified. For instance, the name must match the name used in the @GeneratedValue annotation.
- @GeneratedValue Strategy: Use the appropriate generation type. The strategy should be set to
SEQUENCE
for a sequence generator to function correctly. - Database Sequence Existence: Verify that the database sequence exists. If it doesn’t, the generator can’t obtain a value.
Database Compatibility Issues
Database compatibility can impact how well the JPA sequence generator operates. Be aware of the following:
- Database Type: Ensure the database supports sequences. Some databases, like MySQL (prior to version 8.0), don’t support them.
- Dialect Configuration: Confirm that the correct Hibernate dialect is set in your configuration. An incorrect dialect may lead to sequence generation failures.
- Permissions: Check that the database user has the necessary permissions to access the sequence. Missing permissions can stop the generator from working properly.
Application-Specific Problems
Application-specific problems may also hinder the proper function of your JPA sequence generator. Consider these factors:
- Entity Mappings: Ensure entity classes are correctly annotated. Improper mappings can prevent the JPA provider from recognizing the generator.
- Multiple Instances: If multiple persistent contexts exist, this can lead to unexpected behavior. Use a single context to manage database operations for consistent ID generation.
- Transaction Management: Analyze transaction boundaries. If transactions aren’t managed correctly, it can disrupt the sequence generation process and lead to errors.
By addressing these areas, you can troubleshoot and resolve issues with your JPA sequence generator, ensuring your application’s identifiers function smoothly.
Troubleshooting JPA Sequence Generator Issues
Addressing JPA sequence generator issues starts with methodical troubleshooting. You can resolve common problems by verifying configuration settings, checking database sequences, and debugging your application code.
Verifying Configuration Settings
Verify the configuration settings in your entity class. Ensure your annotations are correctly defined. For example, check the @SequenceGenerator
for the right name, sequence name, and allocation size:
@SequenceGenerator(name = "mySequence", sequenceName = "my_sequence", allocationSize = 1)
@GeneratedValue(strategy = GenerationType.SEQUENCE, generator = "mySequence")
Make sure that the parameters align with your database’s sequence. An incorrect allocation size may lead to gaps in IDs or conflicts when multiple instances try to access the sequence simultaneously.
Checking Database Sequences
Check whether the corresponding database sequence exists. Execute a query in your database management system to confirm:
SELECT * FROM user_sequences WHERE sequence_name = 'MY_SEQUENCE';
If the sequence isn’t present, create it using the appropriate SQL command:
CREATE SEQUENCE my_sequence START WITH 1 INCREMENT BY 1;
Additionally, ensure that your sequence has the right permissions to be accessed by your application. This includes checking user privileges associated with your database.
Debugging Application Code
Debugging application code is crucial for identifying issues in sequence generation. Look for stack traces or logs during entity persist operations. Ensure your transaction management is functioning correctly—if you use multiple transactions, make sure that the sequence isn’t being fetched or cached improperly.
Use logging frameworks like SLF4J or Log4J to help trace ID generation processes. Consider implementing a step-by-step approach to pinpoint the failure location, examining the relevant methods that rely on ID generation.
Following these practical steps can help you diagnose and fix JPA sequence generator issues effectively.
Best Practices for Using JPA Sequence Generators
Using JPA sequence generators effectively can streamline your application’s ID generation process. Following best practices ensures reliability and efficiency.
Proper Configuration
- Define Annotations Clearly: Always use
@SequenceGenerator
and@GeneratedValue
together. Specify parameters likename
,sequenceName
, andallocationSize
accurately to ensure the sequence functions properly. - Validate Database Sequences: Ensure the database sequence defined in
@SequenceGenerator
exists. Use a query to check if the sequence is present in the respective database schema. - Set Allocation Size Smartly: Adjust
allocationSize
based on your application’s needs. A higher value can improve performance but may lead to gaps in IDs if the application shuts down unexpectedly. - Use Correct Data Types: Match the ID type in your JPA entity with the data type in the database sequence. Consistency prevents mismatches that lead to errors.
- Consistent Testing: Write unit tests focusing on the ID generation logic. Use integration tests to verify that the sequence properly generates unique IDs during actual runtime conditions.
- Monitor Sequence Behavior: Add logging to track ID generation. Pay attention to generated IDs to identify any anomalies. For example, check for duplicates or unexpected gaps.
- Check Permissions: Ensure your application user has appropriate permissions to access the database sequence. Inadequate permissions can block ID retrieval, causing failures.
- Validate Performance: Keep an eye on your application’s performance, especially under heavy loads. Use load testing to ensure the sequence generator can handle increased requests without failure.
Implementing these best practices enhances the reliability of JPA sequence generators and supports smooth operations in your application.
Conclusion
Getting your JPA sequence generator to work smoothly can feel like a daunting task but with the right approach you can overcome the hurdles. By paying attention to configuration settings and ensuring everything is aligned with your database’s requirements you can prevent many common issues.
Remember to validate your annotations and test thoroughly to catch any potential problems early. Following the best practices outlined will not only enhance the reliability of your ID generation but also streamline your development process.
With a bit of patience and careful troubleshooting you’ll have your sequence generator running like a charm in no time. Happy coding!
Frequently Asked Questions
What are JPA sequence generators used for?
JPA sequence generators are used to create unique identifiers for entity instances in applications by utilizing database sequences. They ensure data integrity and prevent duplication of IDs, which is crucial for maintaining reliable and consistent data management in various projects.
How do I define a JPA sequence generator?
To define a JPA sequence generator, use the @SequenceGenerator
and @GeneratedValue
annotations in your entity class. Specify parameters like the sequence name and allocation size to ensure that the ID generation process aligns with your database sequence settings.
What are common problems with JPA sequence generators?
Common issues with JPA sequence generators include configuration errors, database compatibility problems, and improper annotations. Additionally, problems may arise from missing database sequences and incorrect entity mappings, which can hinder the unique identifier generation process.
How can I troubleshoot JPA sequence generator issues?
To troubleshoot issues, first verify your annotations in the entity class. Ensure that the @SequenceGenerator
and @GeneratedValue
are correctly defined, check if the database sequence exists, and confirm the sequence has the right permissions. Logging can also help identify problems.
What best practices should I follow for JPA sequence generators?
Best practices include clearly defining annotations, validating database sequences, and ensuring allocation sizes are set appropriately. Additionally, match data types between your JPA entities and database sequences, conduct thorough testing, and monitor sequence behavior to ensure efficient ID generation.
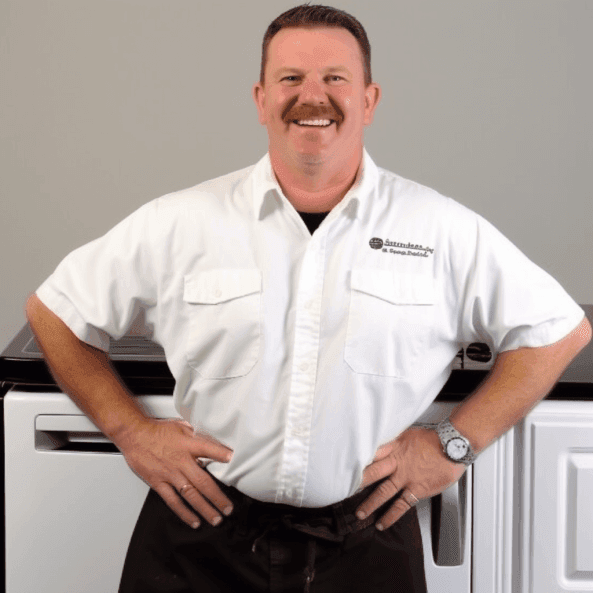
Jake Newman is Appliance Mastery’s expert on refrigeration and cooling systems. With over a decade of experience in the appliance repair industry and certification from NASTeC, Jake is a trusted source of knowledge for homeowners who want to troubleshoot and repair their fridges, freezers, and air conditioning units.